Yo guys !
Im a big big fan of Simcraft, for those of you who don't know what that is heres a link
In Simcraft, to model what the character has to do to achieve the best possible DPS they use a system called Action priority list which in itself is not far from the Behavior Trees used in todays Combat Routines which this Botbase tries to replicate.
Technical stuff, click if you wanna read ^^
1. Botbase with integrated Combat
2. No Movement or Plugin nonsense
3. Classes already implemented are: Fury Warrior, Windwalker Monk, Combat Rogue, Subtlety Rogue, Survival Hunter
4. 3 Hotkeys to steer Execution, Cooldowns and AOE Usage.
5. Optimized Rotations, Simcraft APL Style
6. Highly performant via FrameLock and Caching, one iteration of the combat Routine takes less than 10ms
7. 15 Actions per Second (you can increase this but why
?)
https://github.com/insaneKane/simpl
Heres the Github, you dont need GIT you can just download the ZIP.
Remember, this goes into your Honorbuddy/Bots Folder
PS:
Combat Rogues, the Bot will enable Bladeflurry once there are two targets but you will have to turn it off manually since its hard to programmatically decide when it should be turned off.
You can now easily create your own Rotations ! Check out the profile folder for (mostly working XD) examples
Hunter / Warrior / Combat/Assa Rogue / Moonkin / Deathknight / Monk should work out of the Box
Mage works but i dont know if it works correctly
I dont have a warlock to test things, the apls seem super complicated though so it probably has some bugs
Shaman should work as well
Feral / Sub Rogue / Shadow Priest default profiles do not work, you will need to write your own.
Again check the profiles folder for examples its pretty easy
You can aso use profiles you import from simcraft, simply put your imported character in a simc file inside the profiles folder
Profiles can now be found under Honorbuddy/Simcraft Profiles, if you dont have that folder you can create it or the bot will
Profile Pack:
View attachment Profiles.zip
Its better if you Simcraft your character and use those though
Im a big big fan of Simcraft, for those of you who don't know what that is heres a link
In Simcraft, to model what the character has to do to achieve the best possible DPS they use a system called Action priority list which in itself is not far from the Behavior Trees used in todays Combat Routines which this Botbase tries to replicate.
Technical stuff, click if you wanna read ^^
Heres a small example
So at first i tried to use Singular and modify it so it follows the simcraft rotation but quickly got annoyed by the large overhead and basically having to rethink every single statement and sometimes entire concepts.
I decided to write the whole thing from scratch using Altecs Prot Warrior Routine for some of the basics while trying to allow the User to write Behavior Code as close to simcraft as possible.
Using Dynamics, Caching, LazyInitialisation and some Maneuvering it was possible to enable very special C# code thats very close to actual Simcraft APL Script.
To implement a new Class/Specc Combo you simply need to write a single Function, heres the Combat Rogue for example:
The Botbase will automatically select the function based on the properties you see above the method head.
There are 3 Hotkeys that you can define in the Bot Settings, Cooldowns, AOE and Execution.
Execution is to stop the bot completely, nothing will be done
cooldowns_enabled and aoe_enabled need to be checked by the APL itself to enable the player to for example to block AOE when boss mechanics call for it.
Code:
actions+=/arcane_torrent,if=rage<rage.max-40
actions+=/call_action_list,name=single_target,if=active_enemies=1
actions.single_target=bloodbath
actions.single_target+=/recklessness,if=target.health.pct<20
So at first i tried to use Singular and modify it so it follows the simcraft rotation but quickly got annoyed by the large overhead and basically having to rethink every single statement and sometimes entire concepts.
I decided to write the whole thing from scratch using Altecs Prot Warrior Routine for some of the basics while trying to allow the User to write Behavior Code as close to simcraft as possible.
Using Dynamics, Caching, LazyInitialisation and some Maneuvering it was possible to enable very special C# code thats very close to actual Simcraft APL Script.
To implement a new Class/Specc Combo you simply need to write a single Function, heres the Combat Rogue for example:
Code:
[Behavior(WoWClass.Rogue, WoWSpec.RogueCombat, WoWContext.PvE)]
public void GenerateCombatPvEBehavior()
{
actions += Cast(Preparation, ret => !buff[Vanish].up && cooldown[Vanish].remains > 30);
actions += UseItem(13, ret => cooldowns_enabled && melee_range);
actions += Cast(Blade_Flurry, ret => (active_enemies >= 2 && !buff[Blade_Flurry].up));
actions += Cast(Ambush);
actions += Cast(Vanish,ret =>cooldowns_enabled && Time > 10 &&(combo_points < 3 || (talent[Anticipation].enabled && anticipation_charges < 3) ||(combo_points < 4 || (talent[Anticipation].enabled && anticipation_charges < 4))) &&((talent[Shadow_Focus].enabled && buff[Adrenaline_Rush].down && energy < 90 && energy >= 15) ||(talent[Subterfuge].enabled && energy >= 90) ||(!talent[Shadow_Focus].enabled && !talent[Subterfuge].enabled && energy >= 60)));
actions += Cast(Slice_and_Dice,ret =>buff[Slice_and_Dice].remains < 2 ||((target.time_to_die > 45 && combo_points == 5 && buff[Slice_and_Dice].remains < 12) &&buff[Deep_Insight].down));
actions += CallActionList("adrenaline_rush",ret => target.melee_range && (energy < 35 || bloodlust_up) && cooldown[Killing_Spree].remains > 10);
actions += CallActionList("killing_spree",ret =>(energy < 40 || (bloodlust_up) || BloodlustRemains > 20) && buff[Adrenaline_Rush].down &&(!talent[Shadow_Reflection].enabled || cooldown[Shadow_Reflection].remains > 30 ||buff[Shadow_Reflection].remains > 3));
actions += CallActionList("generator",ret =>combo_points < 5 || !dot[Revealing_Strike].Ticking ||(talent[Anticipation].enabled && anticipation_charges <= 4 && buff[Deep_Insight].down));
actions += CallActionList("finisher",ret =>combo_points == 5 && dot[Revealing_Strike].Ticking &&(buff[Deep_Insight].up || !talent[Anticipation].enabled ||(talent[Anticipation].enabled && anticipation_charges >= 4)));
actions.adrenaline_rush += Cast(Adrenaline_Rush, ret => cooldowns_enabled);
actions.killing_spree += Cast(Killing_Spree, ret => cooldowns_enabled);
actions.generator += Cast(Revealing_Strike,ret =>(combo_points == 4 && dot[Revealing_Strike].Remains < 7.2 &&(target.time_to_die > dot[Revealing_Strike].Remains + 7.2)) || !Ticking);
actions.generator += Cast(Sinister_Strike, ret => dot[Revealing_Strike].Ticking);
actions.finisher += Cast(Death_from_Above);
actions.finisher += Cast(Eviscerate);
}
The Botbase will automatically select the function based on the properties you see above the method head.
There are 3 Hotkeys that you can define in the Bot Settings, Cooldowns, AOE and Execution.
Execution is to stop the bot completely, nothing will be done

cooldowns_enabled and aoe_enabled need to be checked by the APL itself to enable the player to for example to block AOE when boss mechanics call for it.
1. Botbase with integrated Combat
2. No Movement or Plugin nonsense
3. Classes already implemented are: Fury Warrior, Windwalker Monk, Combat Rogue, Subtlety Rogue, Survival Hunter
4. 3 Hotkeys to steer Execution, Cooldowns and AOE Usage.
5. Optimized Rotations, Simcraft APL Style
6. Highly performant via FrameLock and Caching, one iteration of the combat Routine takes less than 10ms
7. 15 Actions per Second (you can increase this but why

https://github.com/insaneKane/simpl
Heres the Github, you dont need GIT you can just download the ZIP.
Remember, this goes into your Honorbuddy/Bots Folder

PS:
Combat Rogues, the Bot will enable Bladeflurry once there are two targets but you will have to turn it off manually since its hard to programmatically decide when it should be turned off.
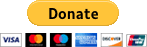
You can now easily create your own Rotations ! Check out the profile folder for (mostly working XD) examples

Hunter / Warrior / Combat/Assa Rogue / Moonkin / Deathknight / Monk should work out of the Box
Mage works but i dont know if it works correctly
I dont have a warlock to test things, the apls seem super complicated though so it probably has some bugs
Shaman should work as well
Feral / Sub Rogue / Shadow Priest default profiles do not work, you will need to write your own.
Again check the profiles folder for examples its pretty easy
You can aso use profiles you import from simcraft, simply put your imported character in a simc file inside the profiles folder
Profiles can now be found under Honorbuddy/Simcraft Profiles, if you dont have that folder you can create it or the bot will

Profile Pack:
View attachment Profiles.zip
Its better if you Simcraft your character and use those though

Last edited: